Show Wallet
In this guide, you will learn how to programmatically display the built-in Arcana wallet in the context of an authenticated user, after integrating a Web3 app with the Arcana Auth SDK.
Prerequisites
-
Use the Arcana Developer Dashboard to register the app and obtain a unique Client ID required for integrating the app with the Arcana Auth SDK.
-
Follow the instructions to configure authentication providers before integrating the app with the Arcana Auth SDK.
-
Use the appropriate integration method as per the app type and integrate the app with the Arcana Auth SDK.
-
Add code in the integrated app to onboard users. The Web3 wallet operations can be invoked programmatically in an app only in the context of an authenticated user.
Steps
Make sure you have addressed the prerequisites before adding code to invoke any Web3 wallet operations supported by the Arcana wallet. After that, plug in the necessary code to set up requisite hooks for JSON/RPC standard Ethereum calls.
// Assuming Auth SDK is integrated and initialized
try {
provider = auth.provider
const connected = await auth.isLoggedIn()
console.log({ connected })
setHooks()
} catch (e) {
// Handle exception case
}
// setHooks: Manage chain or account switch in Arcana wallet
function setHooks() {
provider.on('connect', async (params) => {
console.log({ type: 'connect', params: params })
const isLoggedIn = await auth.isLoggedIn()
console.log({ isLoggedIn })
})
provider.on('accountsChanged', (params) => {
//Handle
console.log({ type: 'accountsChanged', params: params })
})
provider.on('chainChanged', async (params) => {
console.log({ type: 'chainChanged', params: params })
})
}
Display Wallet
A user must be successfully authenticated before calling the showWallet()
function in the context of an app that is integrated with the Arcana Auth SDK.
try {
await auth.showWallet()
} catch (e) {
// Handle exception case
}
If the Arcana wallet is not already displayed in the context of the application, showWallet()
will bring up the following Arcana wallet UI screen. If the Arcana wallet is already displayed in the minimized state before the `showWallet()
function is called, then it will cause the minimized wallet state to change into the maximized Arcana wallet UI.
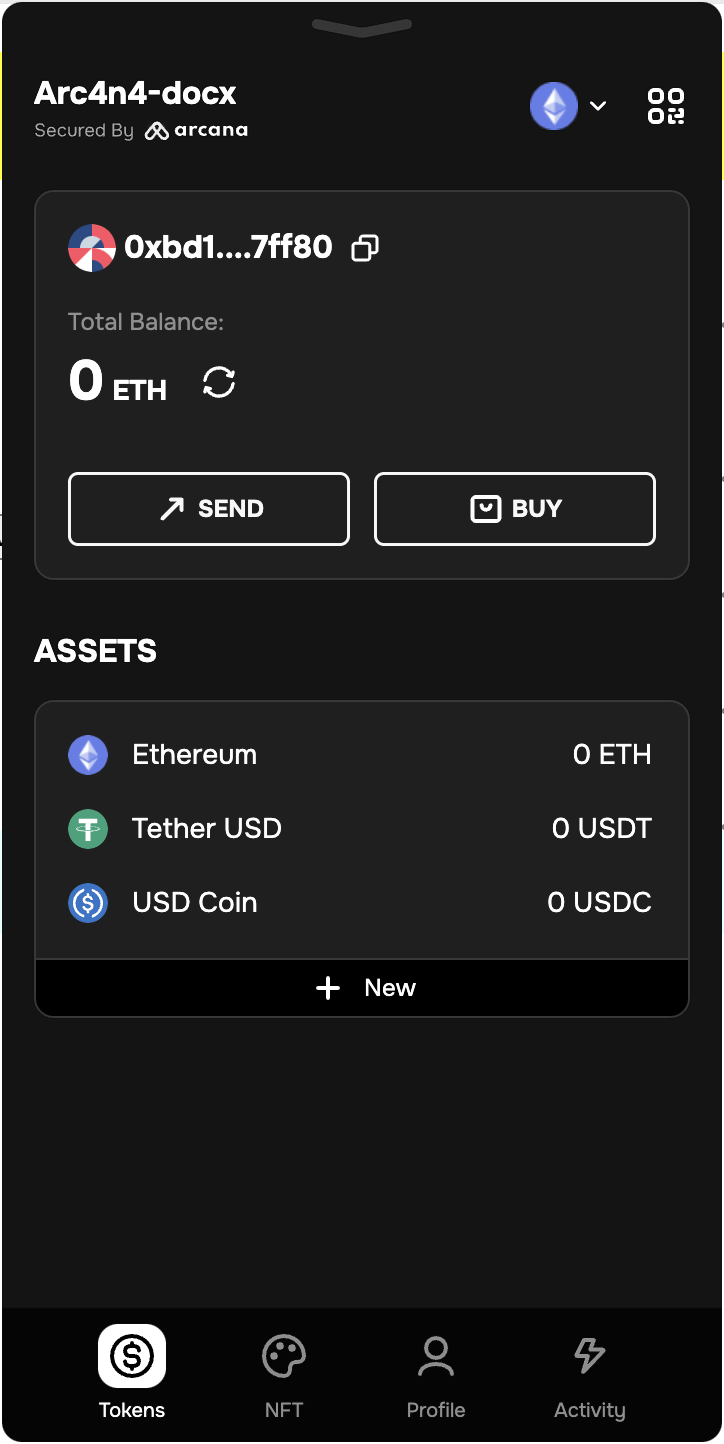
That is all!
The Web3 app is all set to display the built-in Arcana wallet to authenticated users.
An-note
If the AuthProvider
is configured with the default alwaysVisible=true
setting, the built-in Arcana wallet will be automatically displayed in the minimized form when the user logs in. If showWallet()
is called or if the user clicks the minimized wallet UI, then the maximized wallet UI is displayed.
If the alwaysVisible=false
setting is selected by the app developer, then the built-in Arcana wallet is not displayed automatically once the user logs in. It shows up only when a blockchain transaction is triggered. Developers can also bring up the Arcana wallet UI for authenticated users by making an explicit call to the showWallet()
function. Note that the AuthProvider
must be initialized and the user authenticated before the Arcana wallet is displayed.
What's Next?
After registering the app, configuring authentication providers, integrating the Arcana Auth SDK with the app and onboarding users, developers can further add code in the app to sign blockchain transactions, send and receive native, ERC20, or custom tokens, and other Web3 wallet operations.
For a complete list of other JSON RPC calls supported by the Arcana wallet, see JSON-RPC Specifications.
See also
- Arcana wallet capabilities
- Configure Arcana wallet visibility
- Send transactions
- Check the wallet account balance
- Watch crypto assets
- Sign transactions
- Arcana Auth SDK Reference Guide