Next.js App Code Sample
You need to first register the app using the Arcana Developer Dashboard: https://dashboard.arcana.network. Then configure authentication providers for onboarding users. After that, install the following packages and integrate the Next.js app.
auth
auth-react
See sample Next.js app source at GitHub. This is a very basic Next.js app that is created using the create-next-app template. It integrates with the Arcana Auth SDK and uses the built-in, plug-and-play login UI to onboard users and allow them to sign blockchain transactions using the Arcana wallet.
Registration & Configuration
The following Arcana Developer Dashboard screen shows the 'Testnet' configuration settings for the Next.js app such that users can onboard it via Google, Twitch, and passwordless login. See configuration settings for details.

After configuring the app, copy and use the Client ID assigned to the app and displayed in the dashboard on the top right of the Arcana Developer Dashboard screen. It will be used during the app integration with the Arcana Auth SDK.
Integrate App
In the sample code, refer to the auth/getArcanaAuth.js
file. It shows how AuthProvider
is instantiated. Use the Client ID assigned to the app earlier.
import { AuthProvider } from "@arcana/auth";
const auth = new AuthProvider(process.env.NEXT_PUBLIC_ARCANA_APP_ID, { //assigned during app registration, see dashboard
theme: "light", //Check SDK Reference Guide for other optional parameters
});
const getAuth = () => {
return auth;
};
Update Next.js App Context
pages/app.js
After integrating the Arcana Auth SDK and instantiating the AuthProvider
, update _app.js
to plug in the AuthProvider
context ProvideAuth
into the Next.js app.
import { getAuth } from "../auth/getArcanaAuth";
import { ProvideAuth } from "../auth/useArcanaAuth";
import Layout from "../components/layout";
import { Sora } from "@next/font/google";
const sora = Sora();
const auth = getAuth();
export default function App({ Component, pageProps }) {
return (
<ProvideAuth provider={auth}>
<Layout className={sora.className}>
<Component {...pageProps} />
</Layout>
</ProvideAuth>
);
}
The Auth context provider ProvideAuth
is implemented in auth/useArcanaAuth.js
. Refer to the code to see how other AuthProvider
methods besides the plug-and-play connect
method can be used to access functions such as logout.
pages/index.js
Next, update index.js
and add code to onboard users via the built-in, plug-and-play login UI and call the connect
function when the app user chooses to click Connect in the app UI.
import React from "react";
import { useArcanaAuth } from "../auth/useArcanaAuth";
import Loader from "../components/loader";
import { Info } from "../components/info";
import styles from "./index.module.css";
export default function IndexPage() {
const { user, connect, isLoggedIn, loading, loginWithSocial, provider } =
useArcanaAuth();
const onConnectClick = async () => {
try {
await connect();
} catch (e) {
console.log(e);
}
};
const onConnect = () => {
console.log("connected");
};
React.useEffect(() => {
provider.on("connect", onConnect);
return () => {
provider.removeListener("connect", onConnect);
};
}, [provider]);
return (
<>
{loading && <Loader secondaryColor="#101010" strokeColor="#101010" />}
{!loading && !isLoggedIn && (
<button className={styles.Btn} onClick={onConnectClick}>
Connect
</button>
)}
{!loading && isLoggedIn && <Info info={user} />}
</>
);
}
Deploy Sample App
After registering, configuring, integrating the app with the Arcana Auth SDK, and adding code to onboard users, it is time to run the sample Next.js app. Here is what you will see when you run the Next.js sample app:
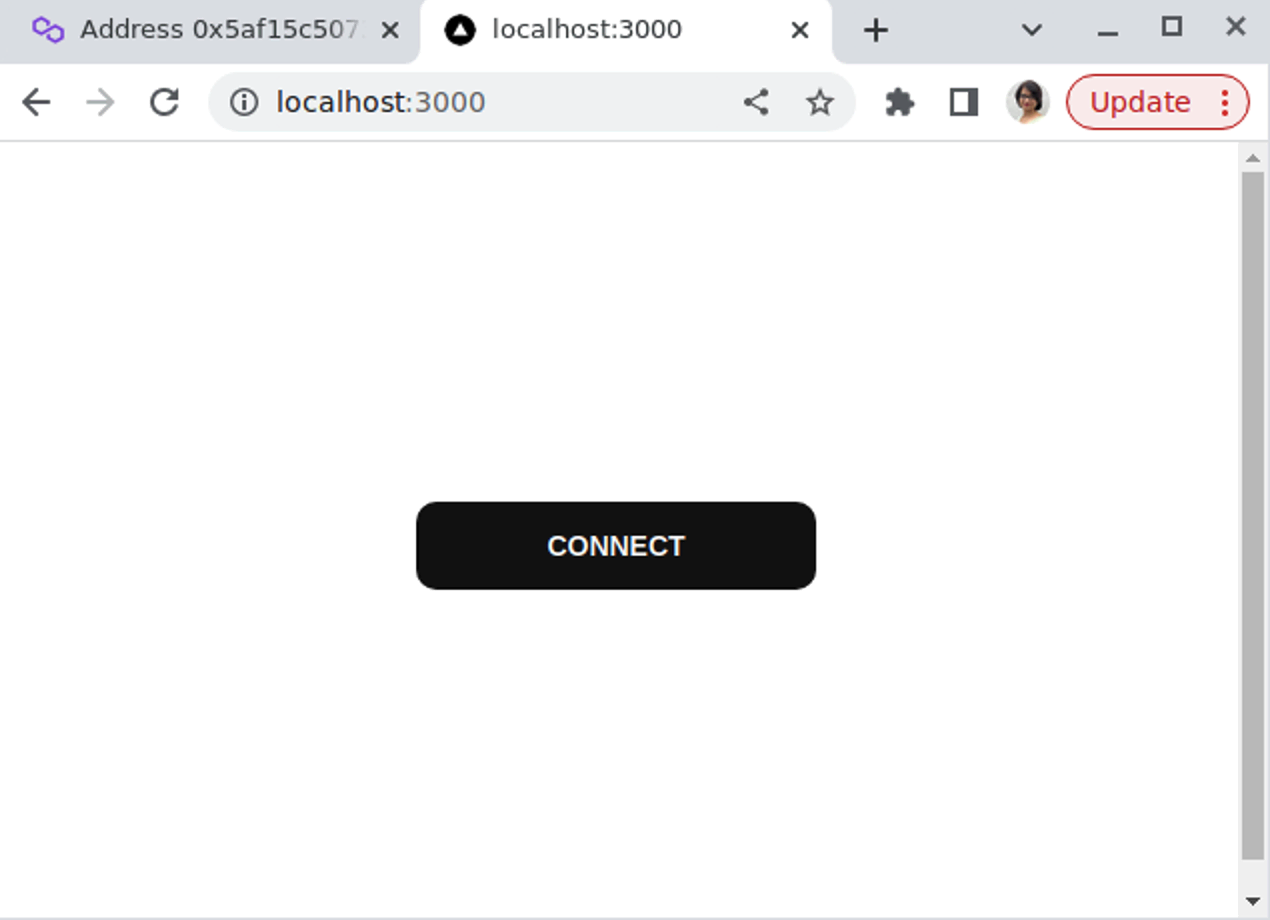
On clicking connect, the Next.js sample app user will see the plug-and-play login screen with options to use Google and Twitch and log into the app. Passwordless authentication is enabled by default.
An-note
In the Next.js sample app, the login options displayed in the "plug-and-play pop-up UI" will only be the ones that have been set up by the developer through the Arcana Developer Dashboard.
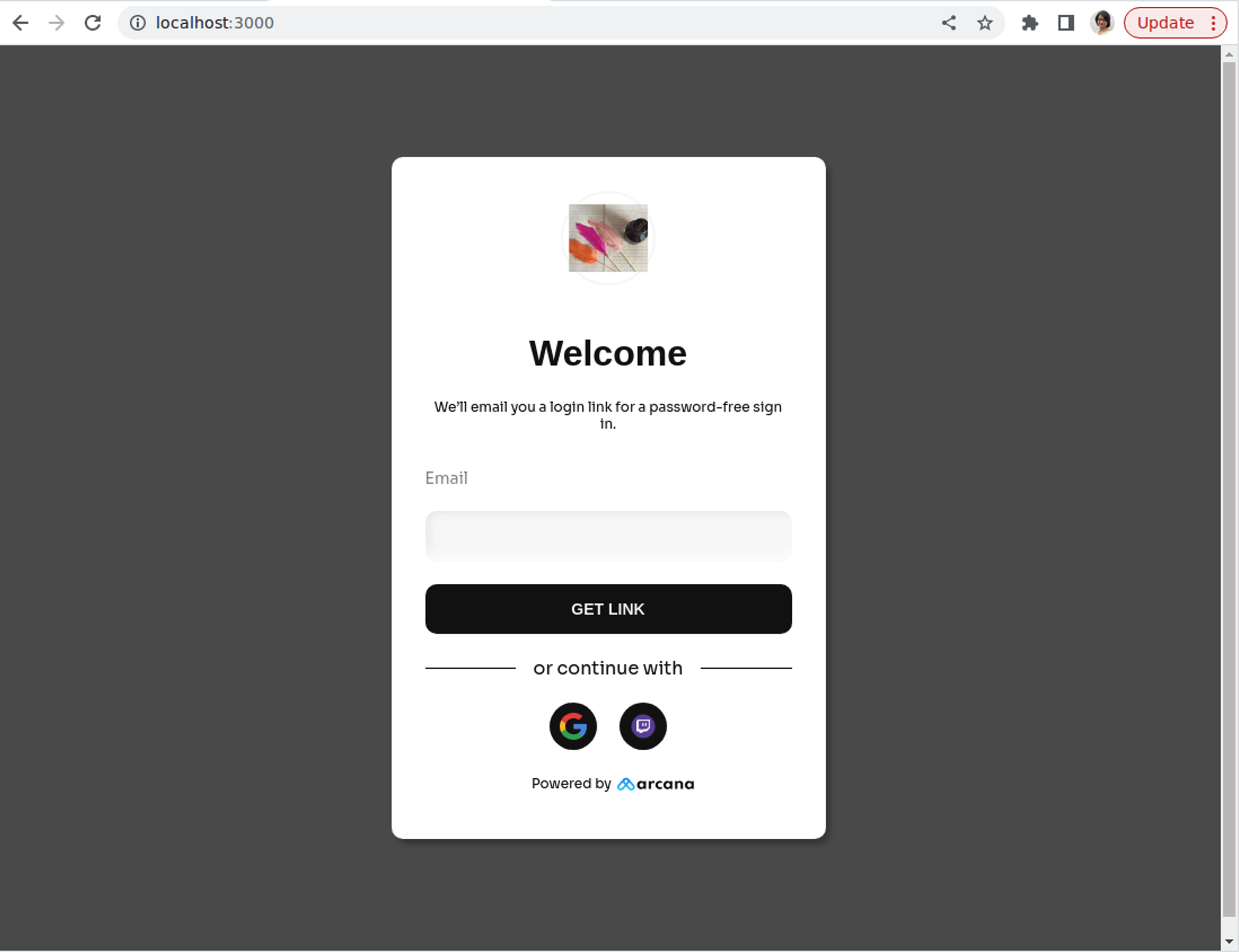
After successful authentication, an app user will see the wallet in its minimized state. On clicking the minimized wallet, the maximized Arcana wallet is displayed. The logo displayed on the maximized wallet can be customized by the app developer through the Arcana dashboard.
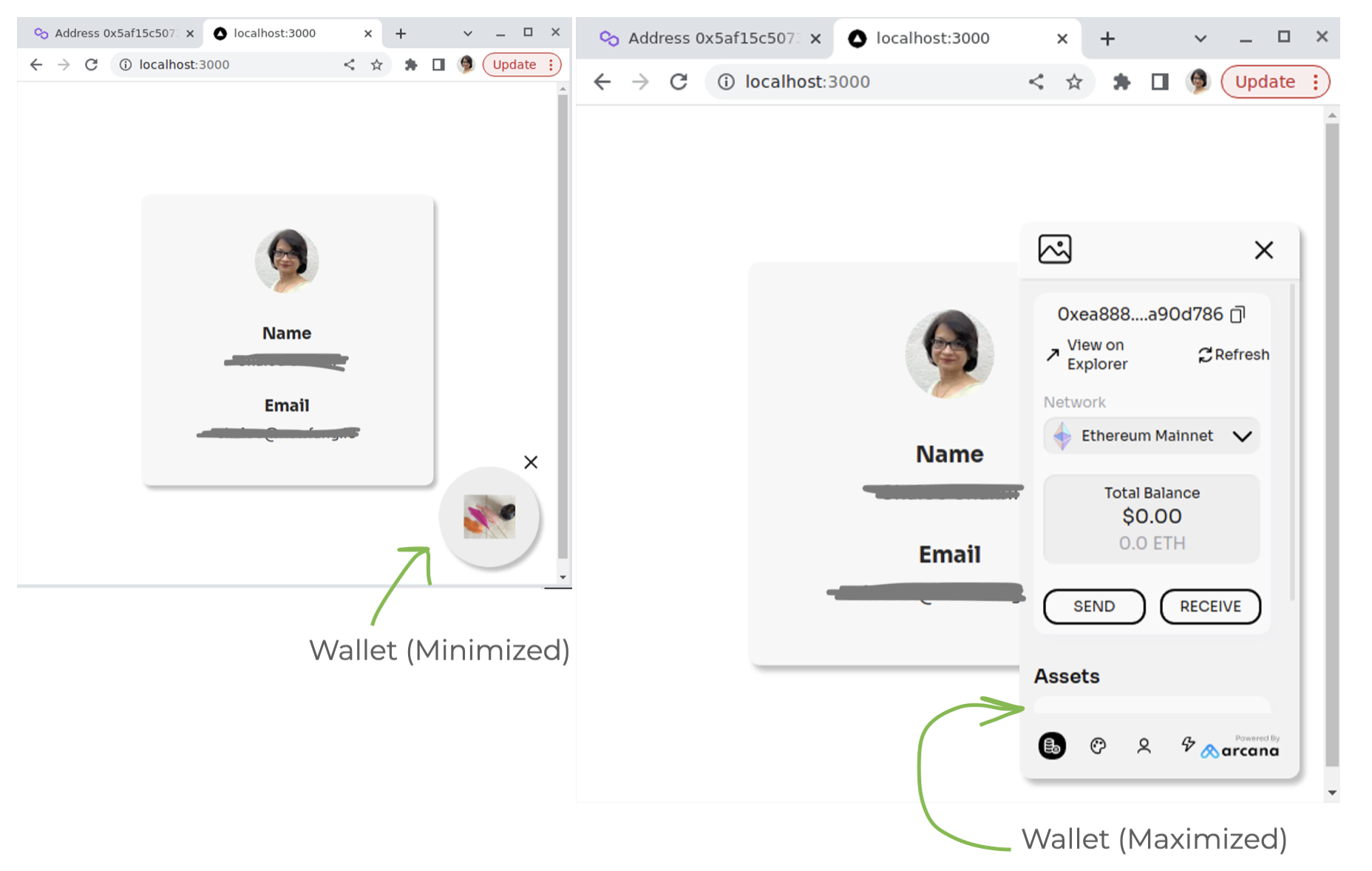
See Arcana wallet User Guide, Arcana wallet Developer's Guide, and the Arcana Developer Dashboard User Guide for more details.
Use the latest Arcana Auth SDK
Check the package.json file in the sample Next.js sources and ensure that you are using the latest Arcana Auth SDK npm release.
The current Arcana Auth SDK release is: v1.0.10