Get Started: HTML/CSS/JS Apps
Estimated time to read: 4 minutes
Integrate 'HTML/CSS/JS' apps with Arcana Auth SDK and onboard users via social login. Enable users to sign blockchain transactions with the in-app Arcana wallet.
Prerequisites
- App must be registered via the Arcana Developer Dashboard: https://dashboard.arcana.network
- Optionally configure auth settings such as social login, wallet user experience, etc.
1. Install
npm install --save @arcana/auth
yarn add @arcana/auth
2. Integrate
import { AuthProvider } from '@arcana/auth'
const auth = new AuthProvider(
"xar_test_445007f942xxxxxxxxxxxxxxxxxx484cAfd2", // App client ID
)
try {
await auth.init()
} catch (e) {
// Handle exception case
}
Initialize First!
The app must use await
until the init()
call is complete, before invoking any of the other Arcana Auth SDK functions listed in Arcana Auth SDK Reference.
Onboard Users
await auth.connect();
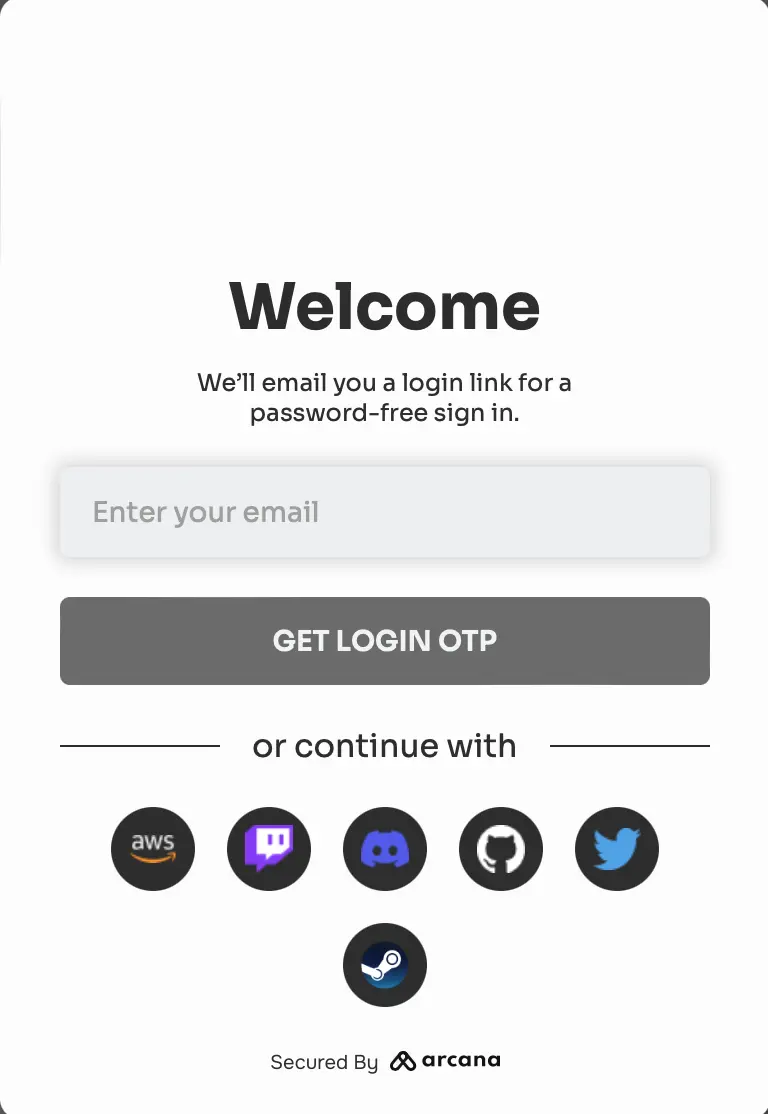
Sign Transactions
Use AuthProvider
, the EIP-1193 provider offered by the SDK, to call supported JSON/RPC functions and Web3 wallet operations in the authenticated user's context.
That's all!
The 'HTML/CSS/JS' app is ready to onboard users and allow them to sign blockchain transactions.
3. Advanced Usage
AuthProvider
Optional Parameters
Besides Client ID input parameter, you can optionally customize these settings in the AuthProvider
constructor:
position
: wallet position within the app context - left
|right
theme
: wallet theme - light
|dark
connectOptions
: compact mode for the built-in plug-and-play login UI - true
|false
connectOptions: {
compact: true // default - false
},
See AuthProvider
constructor parameters for details.
Wallet Customization
Manage the user experience for signing blockchain transactions by configuring SDK usage. Specify the theme, branding settings of the in-app built-in Arcana wallet UI. Use wallet visibility and decide when to display the wallet UI in the app. Configure keyspace and enable the user experience of having the same wallet address across multiple apps integrated with the Arcana Auth SDK.
You can also replace the built-in wallet UI with a custom wallet UI.
Custom Login UI
You can onboard users through a custom login UI instead of the built-in plug-and-play one. See how to use custom login UI and onboard users in a 'HTML/CSS/JS' app.
Compact Login Modal
When using built-in plug-and-play login UI, you can select the compact modal instead of the regular one.
Arcana JWT Token
Upon successful authentication, Arcana Auth SDK returns a unique JWT token to the app called the Arcana JWT Token. App developers can access this token via getUser()
method and refer to the loginToken
field of the UserInfo
object.
Developers can use this token to verify the user and subsequently generate another token for app use if required.
In the future, the Arcana JWT Token will be deprecated. Use userDIDToken
to verify user.
Upon successful authentication, Arcana Auth SDK returns a unique DID token to the app called the Arcana DID Token. App developers can access this token via getUser()
method and refer to the userDIDToken
field of the UserInfo
object.
Developers can use this token to verify the user and subsequently generate another token for app use.
See Also
'HTML/CSS/JS' integration example: See sample-auth-html-css-js
submodule in SDK Example GitHub repository.
Arcana Auth SDK Quick Links