Plug-and-Play Login UI
Estimated time to read: 3 minutes
Use the built-in, plug-and-play login UI modal to quickly onboard users in a 'React/Next.js' app integrated with the Arcana Auth Wagmi SDK.
Custom Login UI
You can onboard users through a custom login UI instead of the built-in plug-and-play one. See how to use custom login UI and onboard users in a 'React/Next.js' app.
Prerequisites
-
Register the Wagmi app and configure SDK usage settings for social login providers, manage app chains and wallet user experience.
-
Install the required SDK packages for 'React/Next.js'.
-
Integrate 'React/Next.js' app, create and initialize the
AuthProvider
.
Steps
import { StrictMode } from "react";
import { createRoot } from "react-dom/client";
import { AuthProvider } from "@arcana/auth";
import { ProvideAuth } from "@arcana/auth-react";
import App from "./App";
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
const provider = new AuthProvider(
"xar_live_d7c88d9b033d100e4200d21a5c4897b896e60063",
{
network: "mainnet",
theme: "light",
connectOptions: {
compact: true,
},
chainConfig: {
chainId: "80001"
}
}
); //See SDK Reference Guide for optional parameters
root.render(
<StrictMode>
<ProvideAuth provider={provider}>
<App />
</ProvideAuth>
</StrictMode>
);
import { Auth } from "@arcana/auth-react";
// Use <Auth/> to use the built-in, plug & play login UI
function App() {
const [theme, setTheme] = React.useState("light");
return (
<div>
<Auth theme={theme} />
</div>
)}
export default App;
import { useAuth } from "@arcana/auth-react";
function App() {
const { loading, isLoggedIn, connect, user } = useAuth()
const onConnectClick = async () => {
try {
await connect(); // Built-in, plug & play login UI
} catch (err) {
console.log({ err });
// Handle error
}
};
if (loading) {
return <p>Loading...</p>;
}
if (!isLoggedIn) {
return (
<button onClick={onConnectClick}>
Login UI (Built-in)
</button>
);
}
}
export default App
The figure below shows the built-in login UI plug-and-play pop-up authentication screen for a test app.
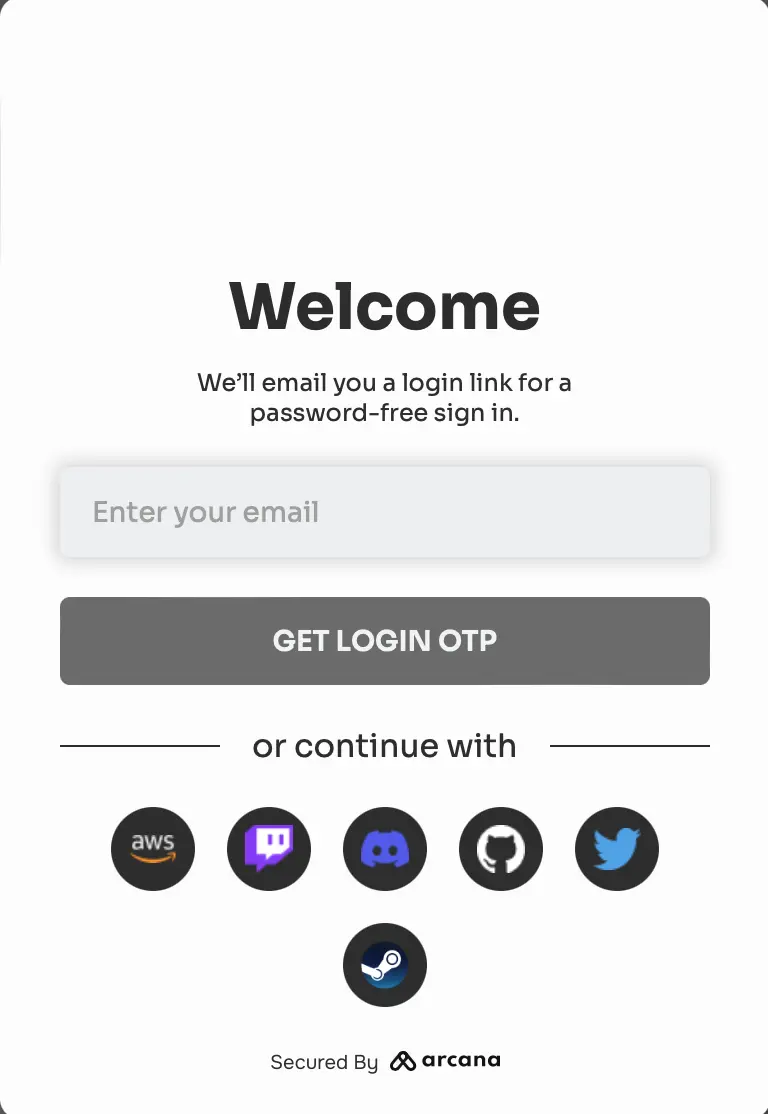
Compact Mode
While creating the AuthProvider
, use connectoOptions
to optionally choose the compact mode for the plug-and-play login UI.
connectOptions: {
compact: true // default - false
},
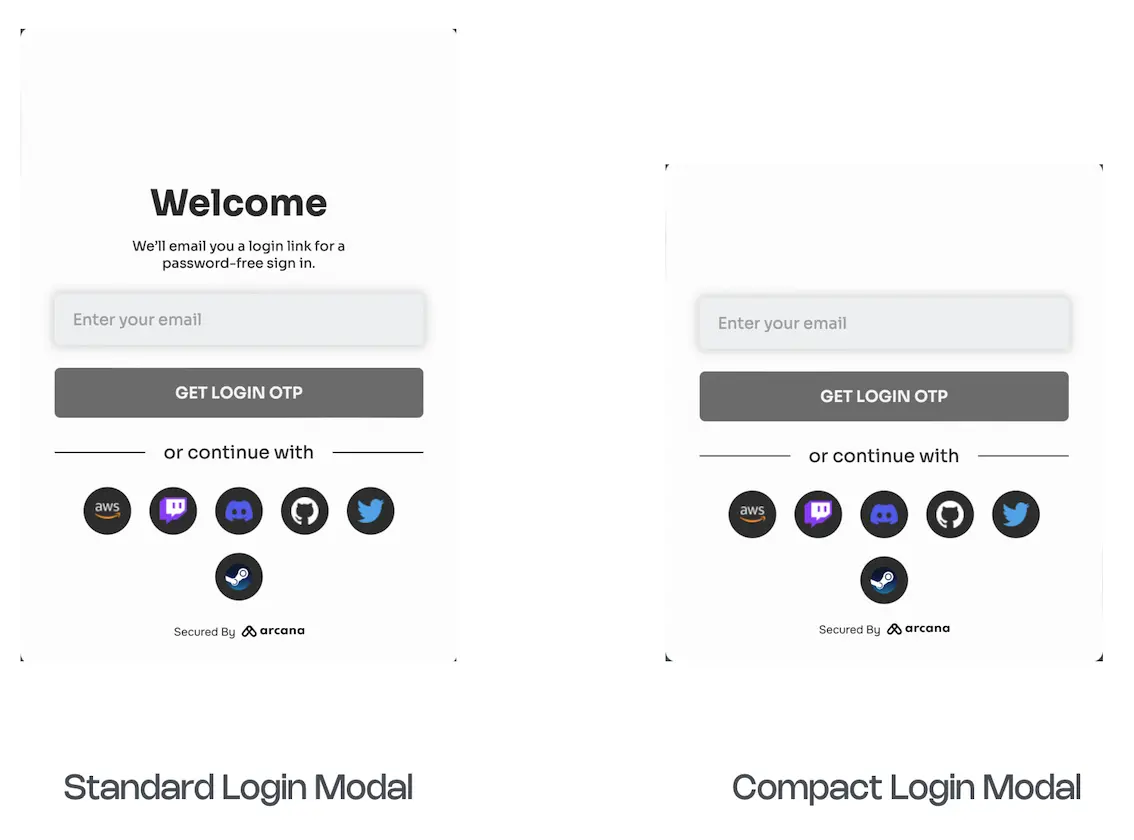
No plug-and-play support for Firebase authentication.
The plug and play feature of the Arcana Auth product is not supported for Firebase. Developers must build a custom login UI and add code to onboard users. For details, see onboarding users via Firebase and custom login UI
No plug-and-play support for Telegram authentication.
The plug and play feature of the Arcana Auth product is not supported for Telegram. Developers must build a custom login UI and add code to onboard users. For details, see [[{{ no such element: dict object['telegram_custom_ui_tag'] }}|onboarding users via Telegram and custom login UI]]
What's Next?
Use AuthProvider
, the EIP-1193 provider offered by the SDK, to call supported JSON/RPC functions and Web3 wallet operations in the authenticated user's context.
See also
'React/Next.js' integration example: See sample-auth-react`,`sample-auth-nextjs
submodule in SDK Example GitHub repository.
Arcana Auth SDK Quick Links
Arcana Auth Wagmi SDK Quick Links